Problem
Let’s start with an example to understand problem. Check the following code:
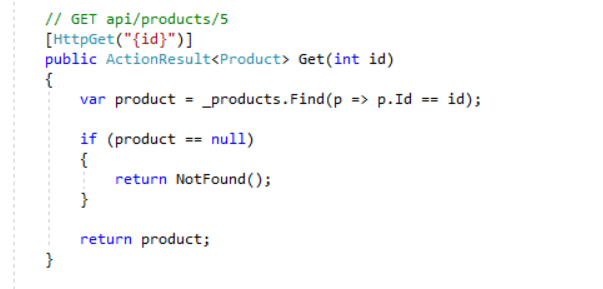
Above API is looking in swagger-ui like following:
As you can see, there is no information that 404 can return, even though method has a NotFound()
method. We can only see info that we request to method with id that is not exist.
When we request to API with not existing product, it returns 404 with un documented warning.
Detect Problem: Analyzers
Analyzers work with controllers annotated with ApiController
introduced in ASP.NET Core 2.1.0, while building on API conventions that is released in ASP.NET Core 2.2.0. To start using this, install the package:
Microsoft.AspNetCore.Mvc.Api.Analyzers
You should see this as warnings (squiggly lines) highlighting return types that aren’t documented as well as warnings in the output. In Visual Studio, this should additionally appear under the “Warnings” tab in the “Error List” dialog. You now have the opportunity to address these warnings using code fixes. Let’s look at the analyzer in action.
Before adding analyzer:
After adding analyzer:
The analyzer identified that the action returned a 404
but did not document it using a ProducesResponseTypeAttribute
. It’s a great way to identify areas of your application that are lacking swagger documentation and correct it.
Fix the Problem
Adding ProducesResponseTypeAttribute
will fix the problem. Check the code:
After adding above attribute, let’s check swagger-ui again:
We know the method can return 404 response. It is documented now.
Conventions
From MSDN: If your controllers follows some common patterns, e.g. they are all primarily CRUD endpoints, and you aren’t already using
ProducesResponseType
orProduces
to document them, you could consider using API conventions. Conventions let you define the most common “conventional” return types and status codes that you return from your action, and apply them to individual actions or controllers, or all controllers in an assembly. Conventions are a substitute to decorating individual actions withProducesResponseType
attributes.By default, ASP.NET Core MVC 2.2 ships with a set of default conventions —
DefaultApiConventions
– that’s based on the controller that ASP.NET Core scaffolds. If your actions follow the pattern that scaffolding produces, you should be successful using the default conventions.
There are 3 ways to apply a convention to a controller action:
- Applying the
ApiConventionType
attribute as an assembly level attribute. This applies the specified convention to all controllers in an assembly.
- Using the
ApiConventionType
attribute on a controller.
- Using
ApiConventionMethod
. This attributes accepts both the type and the convention method.
Authoring conventions
You can write your own conventions. Define a static class to define rules.
And apply this to your controller or action.
And result:
An easy way to get started authoring a custom convention is to start by copying the body of
DefaultApiConventions
and modifying it. Here’s a link to the source of the type: https://raw.githubusercontent.com/aspnet/Mvc/release/2.2/src/Microsoft.AspNetCore.Mvc.Core/DefaultApiConventions.cs
Here is the MSDN article about this topic: https://blogs.msdn.microsoft.com/webdev/2018/08/23/asp-net-core-2-20-preview1-open-api-analyzers-conventions/
Sorce code of examples: https://github.com/alirizaadiyahsi/NetCore22Demos